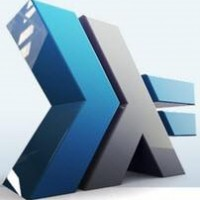
{-
Cube.hs (adapted from cube.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
Cube.hs (adapted from cube.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
This program demonstrates a single modeling transformation, scale and a
single viewing transformation, lookAt. A wireframe cube is rendered.
-}
single viewing transformation, lookAt. A wireframe cube is rendered.
-}
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
import Graphics.UI.GLUT
myInit :: IO ()
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
display :: DisplayCallback
display = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
scalef = scale :: GLfloat -> GLfloat -> GLfloat -> IO ()
color3f (Color3 1 1 1)
loadIdentity — clear the matrix
— viewing transformation
lookAt (Vertex3 0 0 5) (Vertex3 0 0 0) (Vector3 0 1 0)
scalef 1 2 1 — modeling transformation
renderObject Wireframe (Cube 1)
flush
display = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
scalef = scale :: GLfloat -> GLfloat -> GLfloat -> IO ()
color3f (Color3 1 1 1)
loadIdentity — clear the matrix
— viewing transformation
lookAt (Vertex3 0 0 5) (Vertex3 0 0 0) (Vector3 0 1 0)
scalef 1 2 1 — modeling transformation
renderObject Wireframe (Cube 1)
flush
reshape :: ReshapeCallback
reshape size = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
frustum (-1) 1 (-1) 1 1.5 20
matrixMode $= Modelview 0
reshape size = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
frustum (-1) 1 (-1) 1 1.5 20
matrixMode $= Modelview 0
keyboard :: KeyboardMouseCallback
keyboard (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboard _ _ _ _ = return ()
keyboard (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboard _ _ _ _ = return ()
main :: IO ()
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
myInit
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboard
mainLoop
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
myInit
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboard
mainLoop
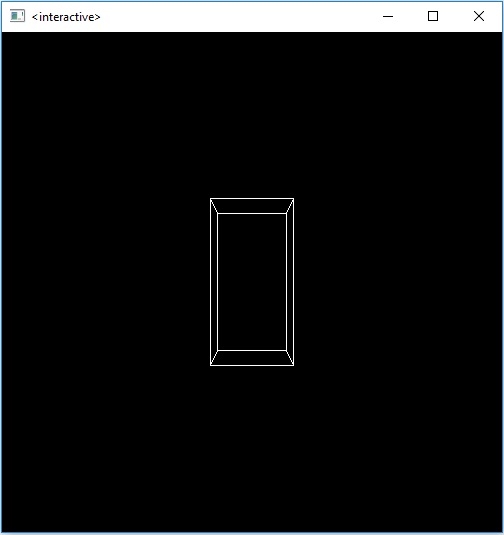
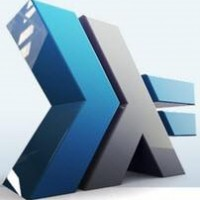
{-
Model.hs (adapted from model.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
Model.hs (adapted from model.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
This program demonstrates modeling transformations.
-}
-}
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
import Graphics.UI.GLUT
myInit :: IO ()
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
drawTriangle :: IO ()
drawTriangle = do
— resolve overloading, not needed in “real” programs
let vertex2f = vertex :: Vertex2 GLfloat -> IO ()
renderPrimitive LineLoop $ do
vertex2f (Vertex2 0 25 )
vertex2f (Vertex2 25 (-25))
vertex2f (Vertex2 (-25) (-25))
drawTriangle = do
— resolve overloading, not needed in “real” programs
let vertex2f = vertex :: Vertex2 GLfloat -> IO ()
renderPrimitive LineLoop $ do
vertex2f (Vertex2 0 25 )
vertex2f (Vertex2 25 (-25))
vertex2f (Vertex2 (-25) (-25))
display :: DisplayCallback
display = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
translatef = translate :: Vector3 GLfloat -> IO ()
scalef = scale :: GLfloat -> GLfloat -> GLfloat -> IO ()
rotatef = rotate :: GLfloat -> Vector3 GLfloat -> IO ()
color3f (Color3 1 1 1)
display = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
translatef = translate :: Vector3 GLfloat -> IO ()
scalef = scale :: GLfloat -> GLfloat -> GLfloat -> IO ()
rotatef = rotate :: GLfloat -> Vector3 GLfloat -> IO ()
color3f (Color3 1 1 1)
loadIdentity
color3f (Color3 1 1 1)
drawTriangle
color3f (Color3 1 1 1)
drawTriangle
lineStipple $= Just (1, 0xF0F0)
loadIdentity
translatef (Vector3 (-20) 0 0)
drawTriangle
loadIdentity
translatef (Vector3 (-20) 0 0)
drawTriangle
lineStipple $= Just (1, 0xF00F)
loadIdentity
scalef 1.5 0.5 1.0
drawTriangle
loadIdentity
scalef 1.5 0.5 1.0
drawTriangle
lineStipple $= Just (1, 0x8888)
loadIdentity
rotatef 90 (Vector3 0 0 1)
drawTriangle
lineStipple $= Nothing
loadIdentity
rotatef 90 (Vector3 0 0 1)
drawTriangle
lineStipple $= Nothing
flush
reshape :: ReshapeCallback
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
let wf = fromIntegral w
hf = fromIntegral h
if w <= h
then ortho (-50) 50 (-50 * hf/wf) (50 * hf/wf) (-1) 1
else ortho (-50 * wf/hf) (50 * wf/hf) (-50) 50 (-1) 1
matrixMode $= Modelview 0
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
let wf = fromIntegral w
hf = fromIntegral h
if w <= h
then ortho (-50) 50 (-50 * hf/wf) (50 * hf/wf) (-1) 1
else ortho (-50 * wf/hf) (50 * wf/hf) (-50) 50 (-1) 1
matrixMode $= Modelview 0
keyboard :: KeyboardMouseCallback
keyboard (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboard _ _ _ _ = return ()
keyboard (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboard _ _ _ _ = return ()
main :: IO ()
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
myInit
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboard
mainLoop
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
myInit
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboard
mainLoop
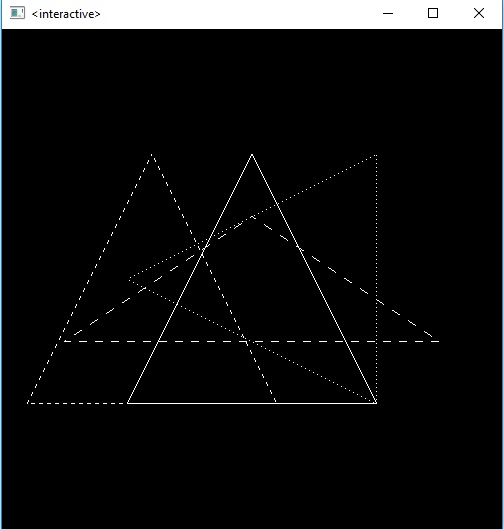
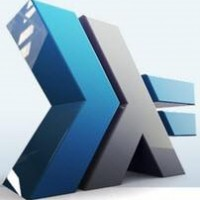
{-
Clip.hs (adapted from clip.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
Clip.hs (adapted from clip.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
This program demonstrates arbitrary clipping planes.
-}
-}
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
import Graphics.UI.GLUT
myInit :: IO ()
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
display :: DisplayCallback
display = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
translatef = translate :: Vector3 GLfloat -> IO ()
rotatef = rotate :: GLfloat -> Vector3 GLfloat -> IO ()
color3f (Color3 1 1 1)
display = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
translatef = translate :: Vector3 GLfloat -> IO ()
rotatef = rotate :: GLfloat -> Vector3 GLfloat -> IO ()
color3f (Color3 1 1 1)
preservingMatrix $ do
translatef (Vector3 0 0 (-5))
translatef (Vector3 0 0 (-5))
— clip lower half — y < 0
clipPlane (ClipPlaneName 0) $= Just (Plane 0 1 0 0)
— clip left half — x < 0
clipPlane (ClipPlaneName 1) $= Just (Plane 1 0 0 0)
clipPlane (ClipPlaneName 0) $= Just (Plane 0 1 0 0)
— clip left half — x < 0
clipPlane (ClipPlaneName 1) $= Just (Plane 1 0 0 0)
rotatef 90 (Vector3 1 0 0)
renderObject Wireframe (Sphere’ 1 20 16)
renderObject Wireframe (Sphere’ 1 20 16)
flush
reshape :: ReshapeCallback
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 60 (fromIntegral w / fromIntegral h) 1 20
matrixMode $= Modelview 0
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 60 (fromIntegral w / fromIntegral h) 1 20
matrixMode $= Modelview 0
keyboard :: KeyboardMouseCallback
keyboard (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboard _ _ _ _ = return ()
keyboard (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboard _ _ _ _ = return ()
main :: IO ()
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
myInit
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboard
mainLoop
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
myInit
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboard
mainLoop
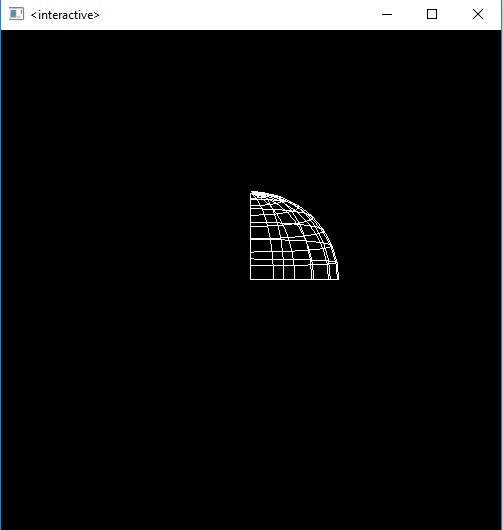
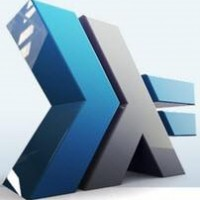
{-
Planet.hs (adapted from planet.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
Planet.hs (adapted from planet.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
This program shows how to composite modeling transformations to draw
translated and rotated models. Interaction: pressing the d and y keys
(day and year) alters the rotation of the planet around the sun.
-}
translated and rotated models. Interaction: pressing the d and y keys
(day and year) alters the rotation of the planet around the sun.
-}
import Data.IORef ( IORef, newIORef )
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
data State = State { year, day :: IORef GLint }
makeState :: IO State
makeState = do
y <- newIORef 0
d <- newIORef 0
return $ State { year = y, day = d }
makeState = do
y <- newIORef 0
d <- newIORef 0
return $ State { year = y, day = d }
myInit :: IO ()
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
display :: State -> DisplayCallback
display state = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
translatef = translate :: Vector3 GLfloat -> IO ()
color3f (Color3 1 1 1)
display state = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let color3f = color :: Color3 GLfloat -> IO ()
translatef = translate :: Vector3 GLfloat -> IO ()
color3f (Color3 1 1 1)
preservingMatrix $ do
renderObject Wireframe (Sphere’ 1 20 16) — draw sun
y <- get (year state)
rotate (fromIntegral y :: GLfloat) (Vector3 0 1 0)
translatef (Vector3 2 0 0)
d <- get (day state)
rotate (fromIntegral d :: GLfloat) (Vector3 0 1 0)
renderObject Wireframe (Sphere’ 0.2 10 8) — draw smaller planet
renderObject Wireframe (Sphere’ 1 20 16) — draw sun
y <- get (year state)
rotate (fromIntegral y :: GLfloat) (Vector3 0 1 0)
translatef (Vector3 2 0 0)
d <- get (day state)
rotate (fromIntegral d :: GLfloat) (Vector3 0 1 0)
renderObject Wireframe (Sphere’ 0.2 10 8) — draw smaller planet
swapBuffers
reshape :: ReshapeCallback
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 60 (fromIntegral w / fromIntegral h) 1 20
matrixMode $= Modelview 0
loadIdentity
lookAt (Vertex3 0 0 5) (Vertex3 0 0 0) (Vector3 0 1 0)
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 60 (fromIntegral w / fromIntegral h) 1 20
matrixMode $= Modelview 0
loadIdentity
lookAt (Vertex3 0 0 5) (Vertex3 0 0 0) (Vector3 0 1 0)
keyboard :: State -> KeyboardMouseCallback
keyboard state (Char c) Down _ _ = case c of
‘d’ -> update day 10
‘D’ -> update day (-10)
‘y’ -> update year 10
‘Y’ -> update year (-10)
‘\27’ -> exitWith ExitSuccess
_ -> return ()
where update angle inc = do
angle state $~ ((`mod` 360) . (+ inc))
postRedisplay Nothing
keyboard _ _ _ _ _ = return ()
keyboard state (Char c) Down _ _ = case c of
‘d’ -> update day 10
‘D’ -> update day (-10)
‘y’ -> update year 10
‘Y’ -> update year (-10)
‘\27’ -> exitWith ExitSuccess
_ -> return ()
where update angle inc = do
angle state $~ ((`mod` 360) . (+ inc))
postRedisplay Nothing
keyboard _ _ _ _ _ = return ()
main :: IO ()
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ DoubleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
state <- makeState
myInit
displayCallback $= display state
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just (keyboard state)
mainLoop
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ DoubleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
state <- makeState
myInit
displayCallback $= display state
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just (keyboard state)
mainLoop
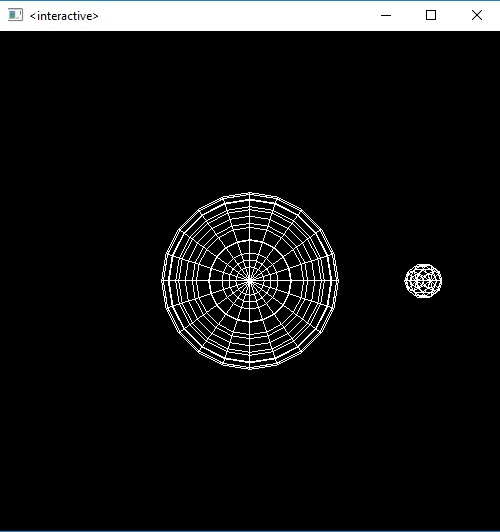
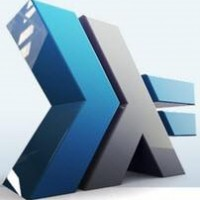
{-
Robot.hs (adapted from robot.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
Robot.hs (adapted from robot.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
This program shows how to composite modeling transformations to draw
translated and rotated hierarchical models. Interaction: pressing the s
and e keys (shoulder and elbow) alters the rotation of the robot arm.
-}
translated and rotated hierarchical models. Interaction: pressing the s
and e keys (shoulder and elbow) alters the rotation of the robot arm.
-}
import Data.IORef ( IORef, newIORef )
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
data State = State { shoulder, elbow :: IORef GLint }
makeState :: IO State
makeState = do
s <- newIORef 0
e <- newIORef 0
return $ State { shoulder = s, elbow = e }
makeState = do
s <- newIORef 0
e <- newIORef 0
return $ State { shoulder = s, elbow = e }
myInit :: IO ()
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
myInit = do
clearColor $= Color4 0 0 0 0
shadeModel $= Flat
display :: State -> DisplayCallback
display state = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let translatef = translate :: Vector3 GLfloat -> IO ()
scalef = scale :: GLfloat -> GLfloat -> GLfloat -> IO ()
preservingMatrix $ do
translatef (Vector3 (-1) 0 0)
s <- get (shoulder state)
rotate (fromIntegral s :: GLfloat) (Vector3 0 0 1)
translatef (Vector3 1 0 0)
preservingMatrix $ do
scalef 2 0.4 1
renderObject Wireframe (Cube 1)
translatef (Vector3 1 0 0)
e <- get (elbow state)
rotate (fromIntegral e :: GLfloat) (Vector3 0 0 1)
translatef (Vector3 1 0 0)
preservingMatrix $ do
scalef 2 0.4 1
renderObject Wireframe (Cube 1)
swapBuffers
display state = do
clear [ ColorBuffer ]
— resolve overloading, not needed in “real” programs
let translatef = translate :: Vector3 GLfloat -> IO ()
scalef = scale :: GLfloat -> GLfloat -> GLfloat -> IO ()
preservingMatrix $ do
translatef (Vector3 (-1) 0 0)
s <- get (shoulder state)
rotate (fromIntegral s :: GLfloat) (Vector3 0 0 1)
translatef (Vector3 1 0 0)
preservingMatrix $ do
scalef 2 0.4 1
renderObject Wireframe (Cube 1)
translatef (Vector3 1 0 0)
e <- get (elbow state)
rotate (fromIntegral e :: GLfloat) (Vector3 0 0 1)
translatef (Vector3 1 0 0)
preservingMatrix $ do
scalef 2 0.4 1
renderObject Wireframe (Cube 1)
swapBuffers
reshape :: ReshapeCallback
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 65 (fromIntegral w / fromIntegral h) 1 20
matrixMode $= Modelview 0
loadIdentity
— resolve overloading, not needed in “real” programs
let translatef = translate :: Vector3 GLfloat -> IO ()
translatef (Vector3 0 0 (-5))
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 65 (fromIntegral w / fromIntegral h) 1 20
matrixMode $= Modelview 0
loadIdentity
— resolve overloading, not needed in “real” programs
let translatef = translate :: Vector3 GLfloat -> IO ()
translatef (Vector3 0 0 (-5))
keyboard :: State -> KeyboardMouseCallback
keyboard state (Char c) Down _ _ = case c of
‘s’ -> update shoulder 5
‘S’ -> update shoulder (-5)
‘e’ -> update elbow 5
‘E’ -> update elbow (-5)
‘\27’ -> exitWith ExitSuccess
_ -> return ()
where update joint inc = do
joint state $~ ((`mod` 360) . (+ inc))
postRedisplay Nothing
keyboard _ _ _ _ _ = return ()
keyboard state (Char c) Down _ _ = case c of
‘s’ -> update shoulder 5
‘S’ -> update shoulder (-5)
‘e’ -> update elbow 5
‘E’ -> update elbow (-5)
‘\27’ -> exitWith ExitSuccess
_ -> return ()
where update joint inc = do
joint state $~ ((`mod` 360) . (+ inc))
postRedisplay Nothing
keyboard _ _ _ _ _ = return ()
main :: IO ()
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ DoubleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
state <- makeState
myInit
displayCallback $= display state
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just (keyboard state)
mainLoop
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ DoubleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
state <- makeState
myInit
displayCallback $= display state
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just (keyboard state)
mainLoop
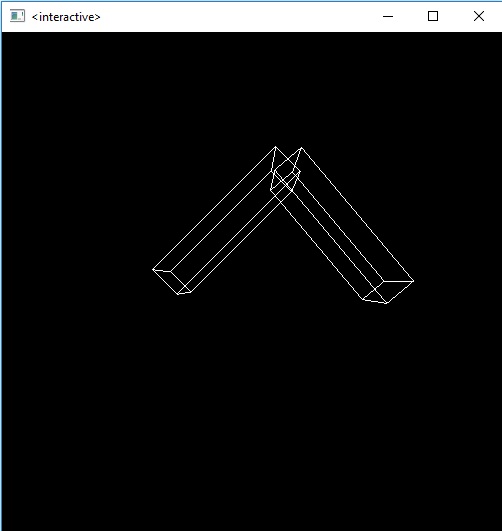
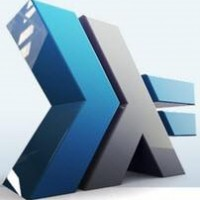
{-
UnProject.hs (adapted from unproject.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
UnProject.hs (adapted from unproject.c which is (c) Silicon Graphics, Inc.)
Copyright (c) Sven Panne 2002-2005 <svenpanne@gmail.com>
This file is part of HOpenGL and distributed under a BSD-style license
See the file libraries/GLUT/LICENSE
When the left mouse button is pressed, this program reads the mouse
position and determines two 3D points from which it was transformed.
Very little is displayed.
-}
position and determines two 3D points from which it was transformed.
Very little is displayed.
-}
import System.Exit ( exitWith, ExitCode(ExitSuccess) )
import Graphics.UI.GLUT
import Graphics.UI.GLUT
display :: DisplayCallback
display = do
clear [ ColorBuffer ]
flush
display = do
clear [ ColorBuffer ]
flush
reshape :: ReshapeCallback
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 45 (fromIntegral w / fromIntegral h) 1 100
matrixMode $= Modelview 0
loadIdentity
reshape size@(Size w h) = do
viewport $= (Position 0 0, size)
matrixMode $= Projection
loadIdentity
perspective 45 (fromIntegral w / fromIntegral h) 1 100
matrixMode $= Modelview 0
loadIdentity
keyboardMouse :: KeyboardMouseCallback
keyboardMouse (MouseButton LeftButton) Down _ (Position x y) = do
v@(_, Size _ h) <- get viewport
mvMatrix <- get (matrix (Just (Modelview 0))) :: IO (GLmatrix GLdouble)
projMatrix <- get (matrix (Just Projection)) :: IO (GLmatrix GLdouble)
let realY = fromIntegral h – y -1
putStrLn (“Coordinates at cursor are (” ++ show x ++”, ” ++ show realY ++ “)”)
w0 <- unProject (Vertex3 (fromIntegral x) (fromIntegral realY) 0) mvMatrix projMatrix v
putStrLn (“World coords at z=0.0 are ” ++ show w0)
w1 <- unProject (Vertex3 (fromIntegral x) (fromIntegral realY) 1) mvMatrix projMatrix v
putStrLn (“World coords at z=1.0 are ” ++ show w1)
keyboardMouse (MouseButton RightButton) Down _ _ = exitWith ExitSuccess
keyboardMouse (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboardMouse _ _ _ _ = return ()
keyboardMouse (MouseButton LeftButton) Down _ (Position x y) = do
v@(_, Size _ h) <- get viewport
mvMatrix <- get (matrix (Just (Modelview 0))) :: IO (GLmatrix GLdouble)
projMatrix <- get (matrix (Just Projection)) :: IO (GLmatrix GLdouble)
let realY = fromIntegral h – y -1
putStrLn (“Coordinates at cursor are (” ++ show x ++”, ” ++ show realY ++ “)”)
w0 <- unProject (Vertex3 (fromIntegral x) (fromIntegral realY) 0) mvMatrix projMatrix v
putStrLn (“World coords at z=0.0 are ” ++ show w0)
w1 <- unProject (Vertex3 (fromIntegral x) (fromIntegral realY) 1) mvMatrix projMatrix v
putStrLn (“World coords at z=1.0 are ” ++ show w1)
keyboardMouse (MouseButton RightButton) Down _ _ = exitWith ExitSuccess
keyboardMouse (Char ‘\27’) Down _ _ = exitWith ExitSuccess
keyboardMouse _ _ _ _ = return ()
main :: IO ()
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboardMouse
mainLoop
main = do
(progName, _args) <- getArgsAndInitialize
initialDisplayMode $= [ SingleBuffered, RGBMode ]
initialWindowSize $= Size 500 500
initialWindowPosition $= Position 100 100
_ <- createWindow progName
displayCallback $= display
reshapeCallback $= Just reshape
keyboardMouseCallback $= Just keyboardMouse
mainLoop
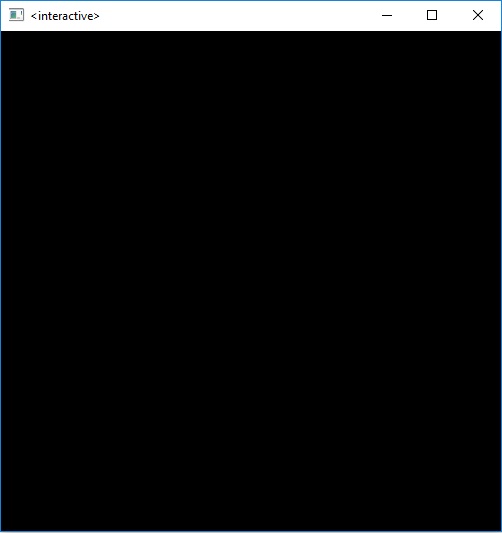
Coordinates at cursor are (210, 281)
World coords at z=0.0 are Vertex3 (-6.627416346087572e-2) 5.1362476682178713e-2 (-0.9999999409914023)
World coords at z=1.0 are Vertex3 (-6.627409697826981) 5.136242515815914 (-99.99989378463076)
World coords at z=0.0 are Vertex3 (-6.627416346087572e-2) 5.1362476682178713e-2 (-0.9999999409914023)
World coords at z=1.0 are Vertex3 (-6.627409697826981) 5.136242515815914 (-99.99989378463076)