Cerință:
Se dau N turnuri și o tablă de șah de dimensiune NxN. Să se găsească toate modalitățile de a aranja toate turnurile astfel încât oricare două turnuri să nu se atace. Două turnuri se atacă dacă se află pe aceeași linie și coloană. Se cere să se afișeze prima soluție în ordine lexicografică și numărul total de soluții.
Indicație de rezolvare:
Aranjarea turnurilor pe tabla de șah este o problema clasica de backtracking. Metoda de rezolvare cu backtracking presupune generarea tuturor soluțiilor si testarea lor daca sunt valide sau nu. Un turn poate fi plasat pe tabla de șah daca pentru fiecare turn aranjat deja, acesta nu se afla pe aceeași coloana sau linie cu niciunul dintre ei.
Implementare:
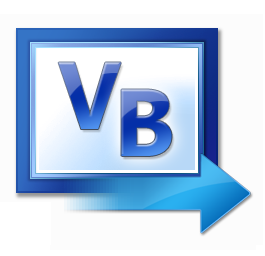
Imports System.Math Module Module1 Dim x(51) As String Dim n, k, nsol As Integer Dim buna As Boolean Function ConditiiContinuare(ByVal k As Integer) Dim atac As Boolean atac = False Dim i As Integer i = 1 While (Not atac And i < k) If (x(i) = x(k)) Then atac = True Else i = i + 1 End If End While Return Not atac End Function Sub ScrieSolutie() nsol = nsol + 1 Console.WriteLine("Solutia " & nsol & " este: ") For i = 1 To n Console.WriteLine(" Asezati tura nr. " & i & " pe linia " & x(i) & " si coloana " & i) Next i Console.ReadLine() End Sub Sub Main() Console.WriteLine("Problema Turelor") Console.Write("Dati numarul n: ") n = Convert.ToInt32(Console.ReadLine()) k = 1 x(k) = 0 nsol = 0 While (k > 0) buna = False While (Not buna And x(k) < n) x(k) = x(k) + 1 If (ConditiiContinuare(k)) Then buna = True End If End While If (buna) Then If (k = n) Then ScrieSolutie() Else k = k + 1 x(k) = 0 End If Else k = k - 1 End If End While End Sub End Module
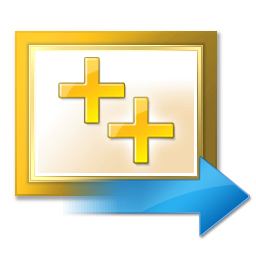
#include "stdafx.h" #include <iostream> #include <conio.h> #include <cmath> using namespace std; int x[51]; int k, n, nsol; bool buna; bool ConditiiContinuare(int k) { // aici testam ca tura k sa nu se atace // pe linie sau pe diagonala cu vreuna // din turele dinaintea sa bool atac=false; int i=1; while (!atac && i<k) if (x[i]==x[k]) atac=true; else i++; return !atac; } void ScrieSolutia() { nsol++; cout<<"Solutie "<<nsol<<" este:\n"; for (int i=1; i<=n; i++) cout<<" Asezati tura nr. "<<i<<" pe linia " <<x[i]<<" si coloana "<<i<<"\n"; getchar(); } int main() { cout<<"Problema turelor\n"; cout<<"Dati numarul n: "; cin>>n; k=1; // plecam cu prima tura x[k]=0; // asezam tura aceasta sub tabla nsol=0; // numarul de solutii while (k>0) // cat timp inca mai avem ture pe tabla { buna=false; // deocamdata tura nu sta bine acolo // cat timp pozitia curenta nu e buna si tura // mai poate fi dusa cu o linie mai sus while (!buna && x[k]<n) { // mut tura mai sus cu un rand/o linie x[k]++; // testez conditiile de continuare k->k+1 if (ConditiiContinuare(k)) buna=true; } if (buna) // daca varianta pentru tura k este buna... if (k==n) // daca am ajuns la ultima tura... ScrieSolutia(); // atunci afisam solutia else // daca nu am ajuns la ultima, { k++; // trecem la urmatoarea tura x[k]=0; // si o asezam sub tabla } else k--; // daca nu (mai) gasim o varianta buna // atunci ne intoarcem la tura anterioara } }
Rezultat:
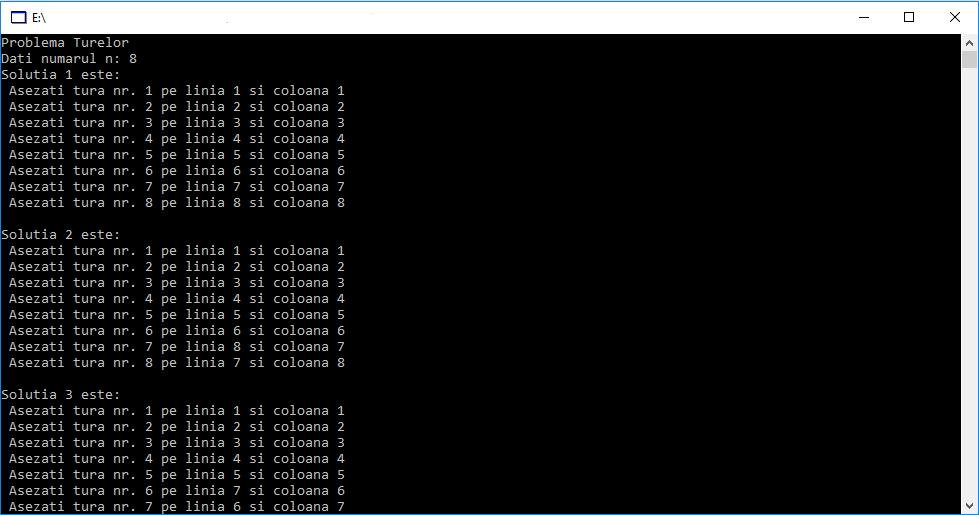
Greetingѕ from Idaho! I’m bored at work so I deided to browse your blog on my iphone durіng lunch break.
I enjoy the information you present hеre and can’t wait tto tak a ⅼοok when I get homе.
І’m surpreised at hοw fast your blog loaded onn
my cell phone .. I’m not even using WIFI, just 3G
.. Anyways, gгeat site!
LikeLiked by 1 person
Just want to say your article is as astounding. The clarity in your post is just excellent and i can assume you’re an expert on this subject.
Fine with your permission let me to grab your RSS feed to keep up to
date with forthcoming post. Thanks a million and please carry on the enjoyable
work.
LikeLike
Some genuinely grand work on behalf of the owner of this site, utterly outstanding content.
LikeLike
Hello there, You have done a fantastic job.
I will definitely digg it and personally recommend to my friends.
I am confident they’ll be benefited from this web site.
LikeLike
I likewise think hence, perfectly written post!
LikeLike
Hey would you mind stating which blog platform you’re
using? I’m looking to start my own blig soon but I’m having a hard
time decoding between BlogEngine/Wordpress/B2evolution and Drupal.
The reason I ask is because your design seems different then ost blogs and I’m looking
for something completely unique. P.S
My apologies forr being off-topic but I had to ask!
LikeLike
You have made some really goodd points there. I checked
on the nett ffor additional information about the issue and
found mos people will go alon with your views on this site.
LikeLike
Hi everyone! Remarkable blog! I adore which you defined Problema Turnurilor – InfoCad Blog.
Like a I’ve used useful brief article with regards to Problema Turnurilor – InfoCad Blog!
Times of explore are over at this moment.
Thank-you, article writer! You’ve undertaken a significant work.
When it comes to myself personally, I personally don’t equally
as crafting make use of doesn’t make any type of entertainment one
more favourable emotions in the event you.
Nonetheless ought to do this one or else I’ll lose out a few investigate .
It is a major concern although there will be be around particular examine services, such as
the one you can visit from mouse pointer that back-link Felicia, the best place professional writers and as a consequence evaluators consider an array
of crafting articles companies to aid whom are
attempting to find tutorial content enable
LikeLike
Hello. I found your blog and this is an extremely well
written post. I’ll bookmark it and return to discover more
of your useful info. Thank you for the post. I’ll really come
back.
LikeLike